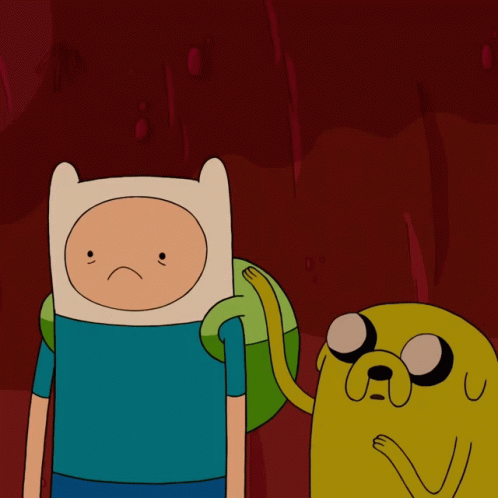
이전글 : [PHP] 게시판 만들기 with MVC - 1부 Migration
이전글 : [PHP] 게시판 만들기 with MVC - 2부 Routing
이전글 : [PHP] 게시판 만들기 with MVC - 3부 View(List & Create)
Controller
웹 사이트 백엔드 기능을 담당한다고 보시면 됩니다. Model과는 통신하며 데이터를 저장, 수정, 삭제를 하며
View에 데이터를 전달하는 역할을 합니다.
제가 이번에 만든 예제는 편의를 위해 View에서 직접 Model에 접근하여 데이터를 가져오는 코드가 존재합니다
하지만 MVC 패턴을 정확히 지키고 싶다면 View는 오직 Controller에서 보낸 데이터만을 사용해야 합니다.
이제 우리는 PostController를 만들어서 글 작성 기능을 만들어봅시다.
우선 Cotroller에서 쓸 편의 기능을 Trait로 만들어줍시다.
bbs/Utils/ControllerUtils.php
<?php
namespace Utils;
trait ControllerUtils {
/**
* 경로로 이동하고 메시지를 출력
* @param $path string 주소
* @param $message string 메시지
* @return void
*/
public function redirect(string $path, string $message)
{
echo "<script>
alert('$message');
location.href='$path';
</script>";
exit();
}
/**
* 이전 페이지로 이동하고 메시지를 출력
* @param $message string 메시지
* @return void
*/
public function redirectBack($message)
{
echo "<script>
alert('$message');
history.back();
</script>";
exit();
}
/**
* json 형식으로 출력 (Ajax 요청에 반환 값)
* @param $data array|object
* @return void
*/
public function echoJson($data){
header('Content-Type: application/json');
echo json_encode($data);
}
/**
* 파라미터가 존재 여부 확인
* @param ...$parameters string 검사할 파라미터
* @return bool
*/
public function parametersCheck(...$parameters): bool
{
foreach ($parameters as $parameter){
if (empty($parameter)){
return false;
}
}
return true;
}
}
Trait를 BaseController에 포함시키고
bbs/Controller/BaseController.php
<?php
namespace Controller;
use Utils\ControllerUtils;
class BaseController{
use ControllerUtils;
}
bbs/Controller/PostController.php
<?php
namespace Controller;
use Model\Post;
class PostController extends BaseController
{
private $post;
// 생성자를 통해 PostModel 객체 생성
public function __construct()
{
$this->post = new Post();
}
/**
* 게시글 생성 기능을 담당
* 데이터 유효성 검사 + Post Model을 통해 데이터 생성을
* @return void
*/
public function create()
{
// POST 데이터 정리
$name = $_POST['name'];
$pw = $_POST['pw'];
$title = $_POST['title'];
$content = $_POST['content'];
// 데이터 유효성 검사
if ($this->parametersCheck($name,$pw,$title,$content)) {
// POST 데이터 생성
if ($this->post->create($name, $pw, $title, $content)) {
$this->redirect('/bbs', '글이 작성되었습니다.');
} else {
$this->redirectBack('글 작성에 실패했습니다.');
}
} else {
$this->redirectBack('입력되지 않은 값이 있습니다.');
}
}
}
여기까지 했다면 Model인 Post에 create가 없어 에러가 발생합니다.
Model
Post 모델에 아래 코드를 추가합시다
bbs/Model/Post.php
<?php
namespace Model;
use PDO;
use PDOException;
class Post extends BaseModel
{
...
/**
* Post 추가
* @param $name
* @param $pw
* @param $title
* @param $content
* @return bool
*/
public function create($name, $pw, $title, $content): bool
{
try {
$hashed_pw = password_hash($pw, PASSWORD_DEFAULT);
$query = "INSERT INTO posts (name, pw, title,content) VALUES (:name, :pw, :title, :content)";
return $this->conn->prepare($query)->execute([
'name' => $name,
'pw' => $hashed_pw,
'title' => $title,
'content' => $content
]);
} catch (PDOException $e) {
error_log($e->getMessage());
return false;
}
}
}
Route
Route 설정도 합시다.
Route 설정을 해야지 bbs/post/create로 post 요청이 들어왔을 때 이를
PostController에 요청을 보내고 PostController는 Post Model로 데이터를 생성할 수 있겠죠.
bbs/Route/PostRoute.php
<?php
namespace Route;
use Controller\PostController;
class PostRoute extends BaseRoute
{
function routing($url): bool
{
$PostController = new PostController();
// 게시글 목록에 대한 라우팅
if ($this->routeCheck($url, "post/list", "GET")) {
return $this->requireView('index');
} else if ($this->routeCheck($url, "post/create", "GET")) {
return $this->requireView('create');
} else if ($this->routeCheck($url, "post/create", "POST")) {
$PostController->create();
return true;
} else {
return false;
}
}
}
결과
이제 아래처럼 글이 추가됩니다.
만약 데이터를 넣지 않으면 "입력하지 않은 값이 있습니다."로 팝업이 뜨고 글 작성 페이지에 머무릅니다.
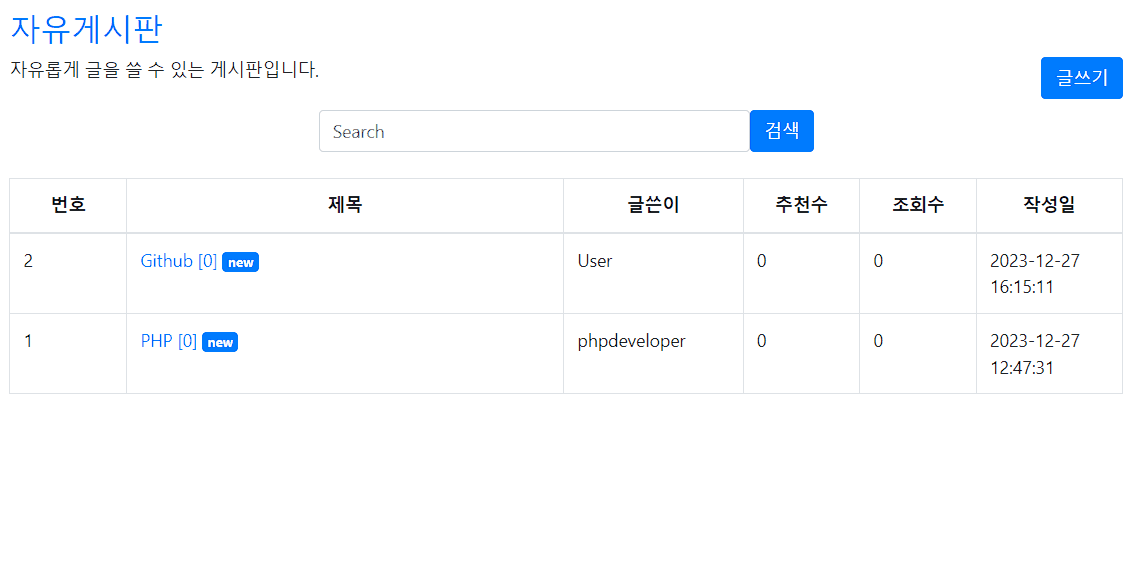
'PHP > Modern PHP' 카테고리의 다른 글
[PHP] 게시판 만들기 with MVC - 6부 Read (0) | 2023.12.27 |
---|---|
[PHP] 게시판 만들기 with MVC - 5부 Pagination (1) | 2023.12.27 |
[PHP] 게시판 만들기 with MVC - 3부 View(List & Create) (1) | 2023.12.27 |
[PHP] 게시판 만들기 with MVC - 2부 Routing (0) | 2023.12.26 |
[PHP] 게시판 만들기 with MVC - 1부 Migration (1) | 2023.12.26 |
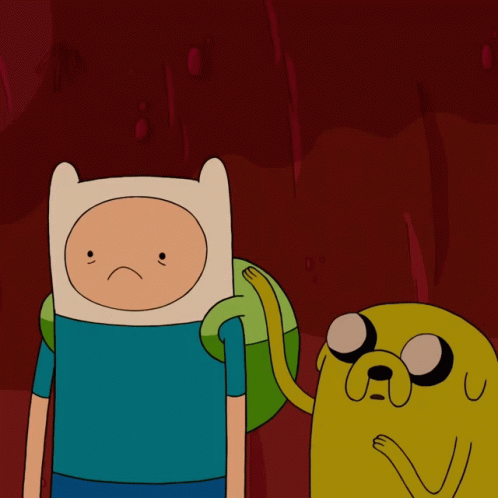
이전글 : [PHP] 게시판 만들기 with MVC - 1부 Migration
이전글 : [PHP] 게시판 만들기 with MVC - 2부 Routing
이전글 : [PHP] 게시판 만들기 with MVC - 3부 View(List & Create)
Controller
웹 사이트 백엔드 기능을 담당한다고 보시면 됩니다. Model과는 통신하며 데이터를 저장, 수정, 삭제를 하며
View에 데이터를 전달하는 역할을 합니다.
제가 이번에 만든 예제는 편의를 위해 View에서 직접 Model에 접근하여 데이터를 가져오는 코드가 존재합니다
하지만 MVC 패턴을 정확히 지키고 싶다면 View는 오직 Controller에서 보낸 데이터만을 사용해야 합니다.
이제 우리는 PostController를 만들어서 글 작성 기능을 만들어봅시다.
우선 Cotroller에서 쓸 편의 기능을 Trait로 만들어줍시다.
bbs/Utils/ControllerUtils.php
<?php
namespace Utils;
trait ControllerUtils {
/**
* 경로로 이동하고 메시지를 출력
* @param $path string 주소
* @param $message string 메시지
* @return void
*/
public function redirect(string $path, string $message)
{
echo "<script>
alert('$message');
location.href='$path';
</script>";
exit();
}
/**
* 이전 페이지로 이동하고 메시지를 출력
* @param $message string 메시지
* @return void
*/
public function redirectBack($message)
{
echo "<script>
alert('$message');
history.back();
</script>";
exit();
}
/**
* json 형식으로 출력 (Ajax 요청에 반환 값)
* @param $data array|object
* @return void
*/
public function echoJson($data){
header('Content-Type: application/json');
echo json_encode($data);
}
/**
* 파라미터가 존재 여부 확인
* @param ...$parameters string 검사할 파라미터
* @return bool
*/
public function parametersCheck(...$parameters): bool
{
foreach ($parameters as $parameter){
if (empty($parameter)){
return false;
}
}
return true;
}
}
Trait를 BaseController에 포함시키고
bbs/Controller/BaseController.php
<?php
namespace Controller;
use Utils\ControllerUtils;
class BaseController{
use ControllerUtils;
}
bbs/Controller/PostController.php
<?php
namespace Controller;
use Model\Post;
class PostController extends BaseController
{
private $post;
// 생성자를 통해 PostModel 객체 생성
public function __construct()
{
$this->post = new Post();
}
/**
* 게시글 생성 기능을 담당
* 데이터 유효성 검사 + Post Model을 통해 데이터 생성을
* @return void
*/
public function create()
{
// POST 데이터 정리
$name = $_POST['name'];
$pw = $_POST['pw'];
$title = $_POST['title'];
$content = $_POST['content'];
// 데이터 유효성 검사
if ($this->parametersCheck($name,$pw,$title,$content)) {
// POST 데이터 생성
if ($this->post->create($name, $pw, $title, $content)) {
$this->redirect('/bbs', '글이 작성되었습니다.');
} else {
$this->redirectBack('글 작성에 실패했습니다.');
}
} else {
$this->redirectBack('입력되지 않은 값이 있습니다.');
}
}
}
여기까지 했다면 Model인 Post에 create가 없어 에러가 발생합니다.
Model
Post 모델에 아래 코드를 추가합시다
bbs/Model/Post.php
<?php
namespace Model;
use PDO;
use PDOException;
class Post extends BaseModel
{
...
/**
* Post 추가
* @param $name
* @param $pw
* @param $title
* @param $content
* @return bool
*/
public function create($name, $pw, $title, $content): bool
{
try {
$hashed_pw = password_hash($pw, PASSWORD_DEFAULT);
$query = "INSERT INTO posts (name, pw, title,content) VALUES (:name, :pw, :title, :content)";
return $this->conn->prepare($query)->execute([
'name' => $name,
'pw' => $hashed_pw,
'title' => $title,
'content' => $content
]);
} catch (PDOException $e) {
error_log($e->getMessage());
return false;
}
}
}
Route
Route 설정도 합시다.
Route 설정을 해야지 bbs/post/create로 post 요청이 들어왔을 때 이를
PostController에 요청을 보내고 PostController는 Post Model로 데이터를 생성할 수 있겠죠.
bbs/Route/PostRoute.php
<?php
namespace Route;
use Controller\PostController;
class PostRoute extends BaseRoute
{
function routing($url): bool
{
$PostController = new PostController();
// 게시글 목록에 대한 라우팅
if ($this->routeCheck($url, "post/list", "GET")) {
return $this->requireView('index');
} else if ($this->routeCheck($url, "post/create", "GET")) {
return $this->requireView('create');
} else if ($this->routeCheck($url, "post/create", "POST")) {
$PostController->create();
return true;
} else {
return false;
}
}
}
결과
이제 아래처럼 글이 추가됩니다.
만약 데이터를 넣지 않으면 "입력하지 않은 값이 있습니다."로 팝업이 뜨고 글 작성 페이지에 머무릅니다.
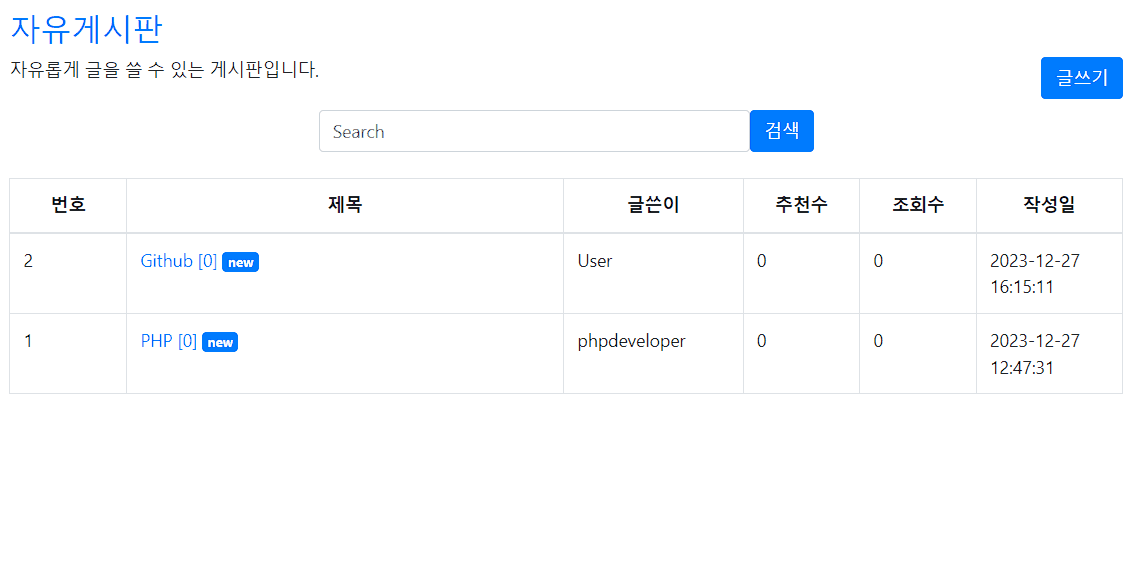
'PHP > Modern PHP' 카테고리의 다른 글
[PHP] 게시판 만들기 with MVC - 6부 Read (0) | 2023.12.27 |
---|---|
[PHP] 게시판 만들기 with MVC - 5부 Pagination (1) | 2023.12.27 |
[PHP] 게시판 만들기 with MVC - 3부 View(List & Create) (1) | 2023.12.27 |
[PHP] 게시판 만들기 with MVC - 2부 Routing (0) | 2023.12.26 |
[PHP] 게시판 만들기 with MVC - 1부 Migration (1) | 2023.12.26 |